How to use an API to create a weather app in only 4 Steps
- Drew Clark
- Feb 3, 2020
- 6 min read
Updated: Feb 5, 2020
Follow this guide to understand what an API is and how to use the Dark Sky API to create a simple weather app. To understand what APIs are, you need to connect to one and play around. It is one of those things where you learn by doing.
Try the Interactive Example below by clicking on Get Weather Data
What is an API?
API - Application Programming Interface
Simply put, it is a way for one application to connect/communicate with another application using a set of commands.
Why would I use an API?
Without them, applications would be pretty limited. Its easier to use someone else's tools than to try create the same functionality. And to be honest, I know that the developers building APIs are probably better than me and have already worked out a lot of kinks.
How to build a simple weather app connected to an API
The Dark Sky API is an API that allows you to look up the weather anywhere on the globe.
Part 1 Create a CodePen account to host and build our web page
Create a CodePen account by clicking here.
Note: We are creating the web page on CodePen for the sake of simplicity and quick learning. We will create a more complex web app with Visual Studio in the next guide.
Create a new pen
Click "Pen" in the top left corner of CodePen.
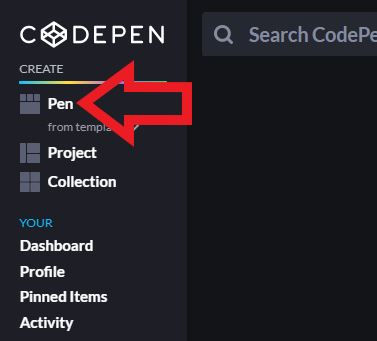
Add example HTML
Copy and past the HTML below into the HTML window, in the Pen you just created.
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
</head>
<body>
<h1>Local Weather App</h1>
<button onclick="getWeatherAPI()">Get Weather Data</button>
<p>Latitude: <span id="latitude"></span></p>
<p>Longitude: <span id="longitude"></span></p>
<p>Timezone: <span id="zone"></span></p>
<p>Temperature: <span id="temp"></span>℉</p>
<p>Windspeed: <span id="windspeed"></span>mph</p>
<p>Condition: <span id="condition"></span></p>
<!--Below is a tag showing this app is using Dark Sky as its data source-->
<h5>Powered by <a href="https://darksky.net/poweredby/">Dark Sky</a></h5>
</body>
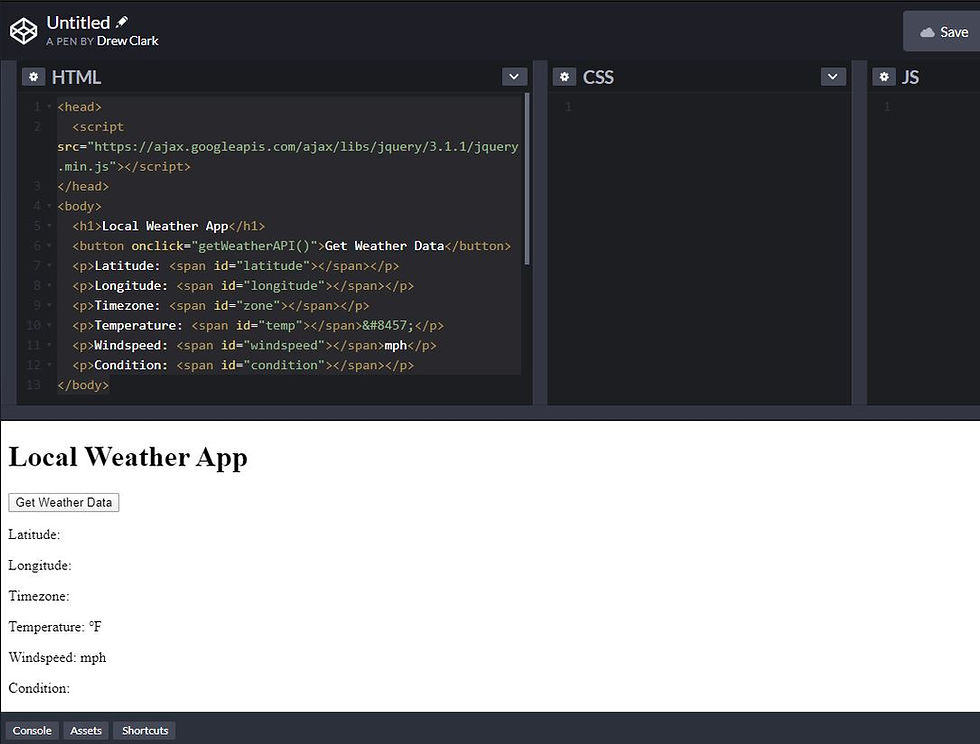
Great you have a button and a few fields where we can display weather information. Lets add the connection to the API to give this web page some functionality.
Part 2 Register a Dark Sky API account to get an API key
Click here to sign up.
A window will open up called Your Account after you register. If it doesn't click console at the top of the screen.
You will see Your Secret Key and a Sample API Call that includes that key.
Note: I don't mind if you see mine because I have no billing information setup on this account and I only use this for simple projects.
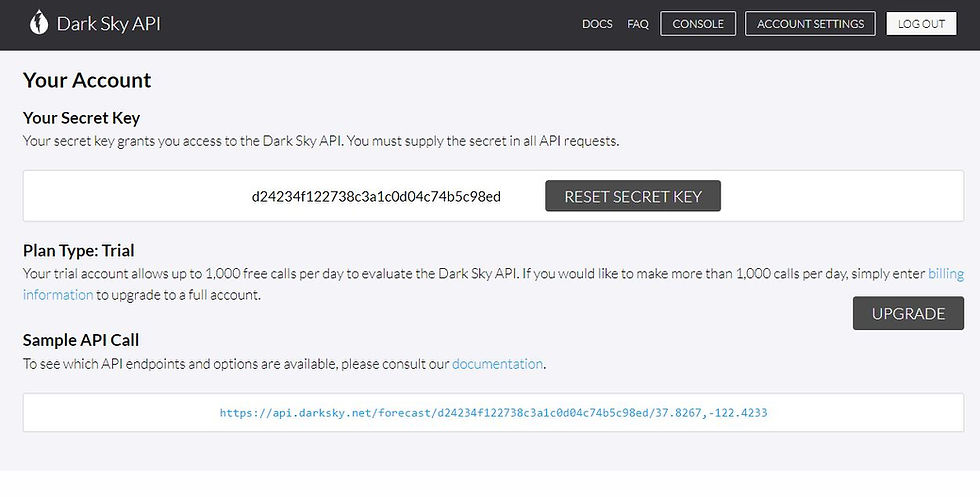
Click on the Sample API call. You should see a ton of text appear on the screen. This is what JSON looks like without formatting. Pretty hard to read, right? Click back to get back to the Dark Sky API console.
Part 3 Add your JavaScript to connect your web app to the API
Copy the JavaScript below into the JS window of your Pen on the right side of the window.
var lati = document.getElementById("latitude");
var long = document.getElementById("longitude");
var zone = document.getElementById("zone");
var temperature = document.getElementById("temp");
var windSpeed = document.getElementById("windspeed");
var condition = document.getElementById("condition");
var secretKey = "PasteKeyHere";
var url = `https://cors-anywhere.herokuapp.com/https://api.darksky.net/forecast/${secretKey}/35.033580,-85.305580`;
function getWeatherAPI () {
fetch(url, {
method: 'GET',
}).then(function(response) {
return response.json()
}).then(function(data) {
lati.innerHTML = data.latitude;
long.innerHTML = data.longitude;
zone.innerHTML = data.timezone;
temperature.innerHTML = Math.round(data.currently.temperature);
windSpeed.innerHTML = data.currently.windSpeed;
condition.innerHTML = data.currently.summary;
});
}

Replace the secret key!
Find the variable called secretKey, inside the quotations, paste your secret key and make sure to not leave any spaces inside the quotations. Your secret key is in the Dark Sky account you created in the second step.
Part 4 Save, Name, and Test your app.
Click save in the top right of the window.
Name your app by clicking the pen icon in the top left corner.
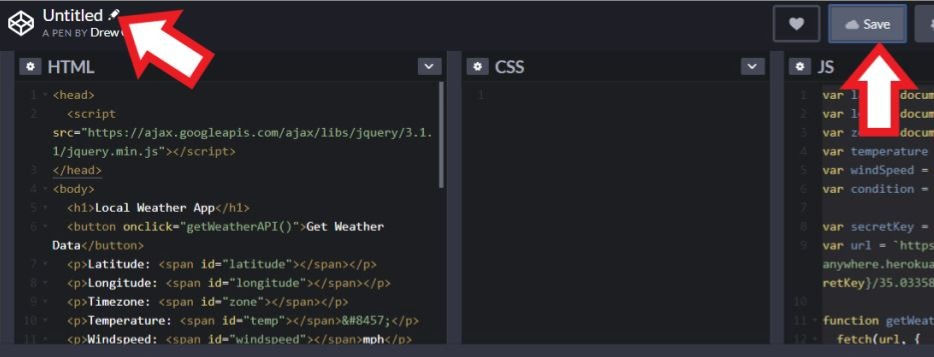
Test It
Click on your Get Weather Data button in the web app to test it!
The local weather data should appear for Chattanooga, TN.

The app works, but we need it to work for your location!
Lets add an input elements for the latitude and longitude so that you can search for weather data anywhere.
Try the Interactive Example below by clicking on Get Weather Data
How to adjust app to have latitude and longitude inputs
Replace HTML with new example code
Remove all your HTML in Codepen and replace it with the HTML below.
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
</head>
<body>
<h1>Local Weather App</h1>
<button onclick="getWeatherAPI()">Get Weather Data</button>
<form>
Latitude: <input type="text" id="latitude" value=40.753742 ><br>
Longitude: <input type="text" id="longitude" value=-73.983569>
</form>
<p>Timezone: <span id="zone"></span></p>
<p>Temperature: <span id="temp"></span>℉</p>
<p>Windspeed: <span id="windspeed"></span>mph</p>
<p>Condition: <span id="condition"></span></p>
</body>
Replace JavaScript
//declare variables for html elements
var zone = document.getElementById("zone");
var temperature = document.getElementById("temp");
var windSpeed = document.getElementById("windspeed");
var condition = document.getElementById("condition");
//function to connect to api and fill in data
function getWeatherAPI () {
var latitude = document.getElementById("latitude").value;
var longitude = document.getElementById("longitude").value;
var secretKey = "d24234f122738c3a1c0d04c74b5c98ed";
var url = `https://cors-anywhere.herokuapp.com/https://api.darksky.net/forecast/${secretKey}/${latitude},${longitude}`;
//request to api
fetch(url, {
method: 'GET',
}).then(function(response) {
return response.json()
}).then(function(data) {
//take the API response and send the data to the html elements
zone.innerHTML = data.timezone;
temperature.innerHTML = Math.round(data.currently.temperature);
windSpeed.innerHTML = data.currently.windSpeed;
condition.innerHTML = data.currently.summary;
});
}
Save your code
Click Save in the top right corner before doing anything else.
Test the app by getting coordinates for a location.
Click here to go to google maps.
Type in a place anywhere in the world.
Click on the map to create a point, notice the coordinates appear at the bottom of the window.
Type those coordinates into your weather app input boxes.
I am using Bryant Park in New York City
40.753742, -73.983569
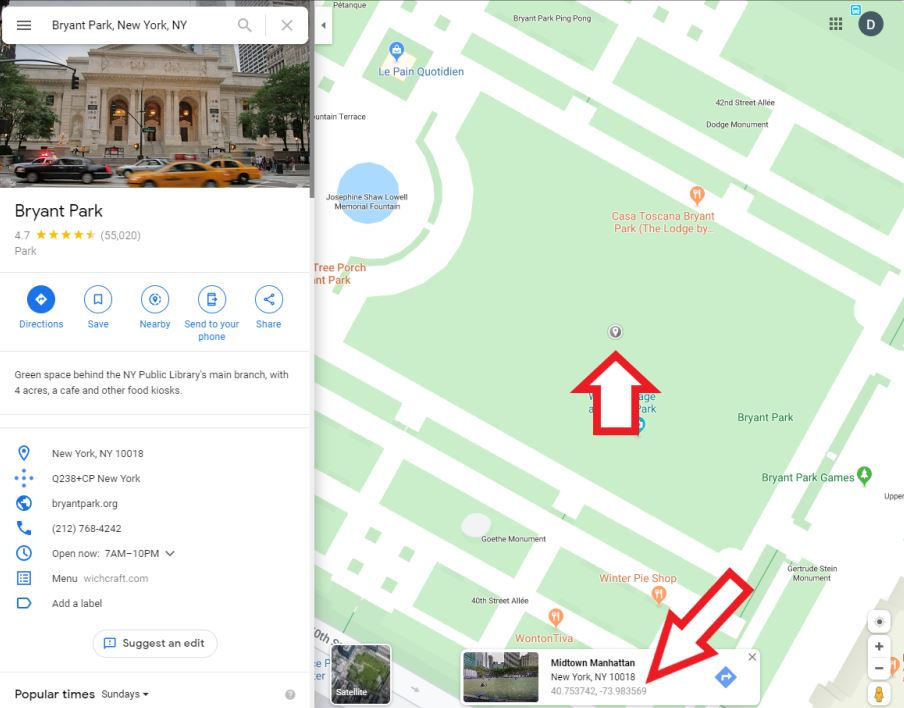
Once you have entered coordinates click your Get Weather Data button to test the app. If the data does not appear, click refresh and try entering the coordinates again.

You finished, but it will not matter unless you understand the code involved so that you can build apps that work for you.Let's break down each piece and see how they work.
How does the HTML work in the example above?
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
</head>
This is used so that our JavsScript file can reference the JQuery library. JQuery takes a lot of common tasks that require many lines of JavaScript code to accomplish, and wraps them into methods that you can call with a single line of code.
<body>
<h1>Local Weather App</h1>
//This is our large label for our web app
<button onclick="getWeatherAPI()">Get Weather Data</button>
//This creates and labels our button and tells the JavaScript which function to call when it is clicked.
<form>
Latitude: <input type="text" id="latitude">
Longitude: <input type="text" id="longitude">
</form>
This form and primarily the input tags, allow us to enter information into our app. We need the ids so that we can get the input values in our JavaScript function.
<p>Timezone: <span id="zone"></span></p>
<p>Temperature: <span id="temp"></span>℉</p>
//See the comment below, but I did want to mention that ℉ is the Degrees Fahrenheit symbol
<p>Windspeed: <span id="windspeed"></span>mph</p>
<p>Condition: <span id="condition"></span></p>
</body>
The remaining items are paragraph items with span tags placed inside them. The span tags and their corresponding ids are used to push the weather data from our JavaScript into the HTML.
How does the JavaScript work in the example above?
var zone = document.getElementById("zone");
var temperature = document.getElementById("temp");
var windSpeed = document.getElementById("windspeed");
var condition = document.getElementById("condition");
The above code is used to create variables and set them equal to the corresponding HTML elements. We do this so we can quickly push our weather data into the HTML elements.
//The below function getWeatherAPI() is the function our button will call when clicked.
function getWeatherAPI () {
//First we grab the input values for lat and long from the HTML form.
var latitude = document.getElementById("latitude").value;
var longitude = document.getElementById("longitude").value;
//We use the following lines to tell the API request that we want weather data for a particular location and to pass our secret key so that it will approve our request.
var secretKey = "d24234f122738c3a1c0d04c74b5c98ed";
//Notice that we are using string interpolation ${} to place our secretKey, lat and long values into the API request.
//Fetch is used to make the API request.
fetch(url, {
method: 'GET',
}).then(function(response) {
return response.json()
// this line above tells the API that we want our data to be returned to us in JSON.
}).then(function(data) {
//the lines below take the API response data and send the data to the html elements.
zone.innerHTML = data.timezone;
temperature.innerHTML = Math.round(data.currently.temperature);
windSpeed.innerHTML = data.currently.windSpeed;
condition.innerHTML = data.currently.summary;
});
}
Next Step
Typing in coordinates sucks, it would be better if we could just type in an address or use our browser to show the api our location.
In the next guide we will use the Google Maps Geocode api.
Please comment and share if you have the time. All feedback is appreciated and helps me learn more about what I could do differently. Thank you for reading.
Comments