Addressing the gap between new JavaScript Syntax and the syntax older web browsers recognize. In this technical guide you will use Babel - A JavaScript library that you can use to convert new, less supported JavaScript (ES6), into an older version (ES5) that is recognized by most modern browsers.
ES6 Syntax Example - We will use a very simple example using const, let, and string interpolation, features of ES6 syntax.

In a modern browser, the console would display My pet Lucky is a Golden Retriever.
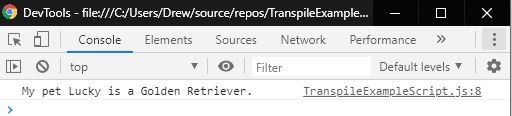
In an old browser we would get errors so we transpile the code into an older version that is supported by more browsers.
Transpile - a specific term for taking source code written in one language and transforming into another language that has a similar level of abstraction.
The code above, if written in ES5 syntax would be as follows.
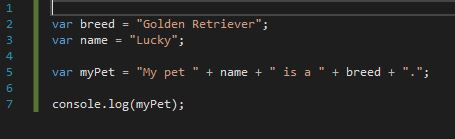
The console will display the same console message above, but it is compatible with both older and modern browsers. Transpiling by hand would be a nightmare, to do it programmatically we use Babel.
Install package.json
Create a folder for this example Project. I made one called TranspileExample.
Inside that folder create another folder called src. Place your script to be transpiled into that folder.
Open up a terminal and navigate to the first folder you created.
Type npm init - This command creates a package.json file in the root directory.
It will ask you to name the package, I used the default file name transpileexample. You can not use capital letters.
Press Enter until the prompt is finished, about 10 times. The final prompt message will say "Is this OK?" press enter.
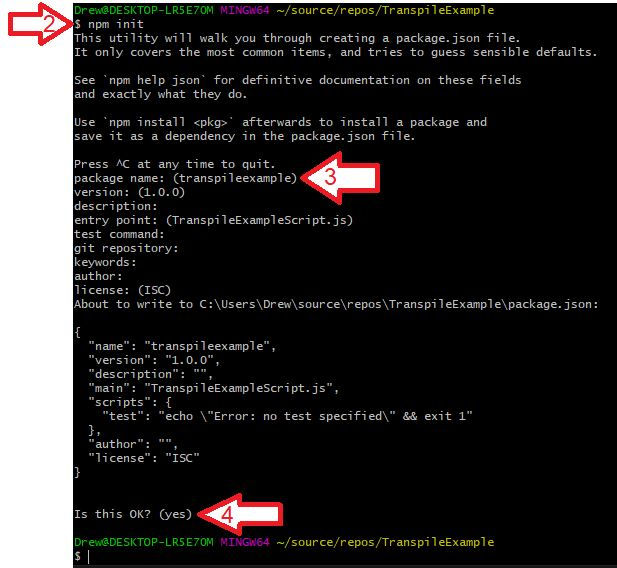
Download & Install Babel
Type npm install babel-cli -D (This command may take a second to run, just wait) This will install the Babel command line package and add it it to the devDependencies property in package.json
Then type npm install babel-preset-env -D (just wait) This will install the Babel preset environment package and add it to the devDependencies property in package.json
Now that you’ve downloaded the Babel packages, you need to specify the version of the source JavaScript code. Type touch .babelrc to create a file that specifies the initial JavaScript version.
Open the newly created file and add the following JavaScript Object into .babelrc. { "presets": ["env"] }
Save the file and close it.
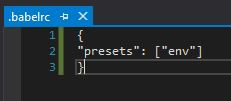
When you run Babel, it looks in .babelrc to determine the version of the initial JavaScript file. In this case, ["env"] instructs Babel to transpile any code from versions ES6 and later.
Babel Source Lib
There’s one last step before we can transpile our code. We need to specify a script in package.json that initiates the ES6+ to ES5 transpilation.
Open the package.json file, there is a property named "scripts" that holds an object for specifying command line shortcuts.
After "test": "echo \"Error: no test specified\" && exit 1" place a comma and press enter.
Type "build": "babel src -d lib" (it should looks like the image below)
Save and close the file
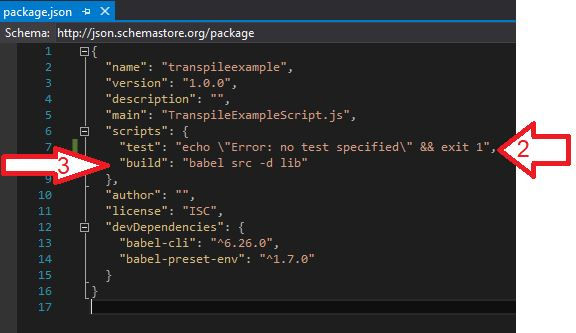
When we run this build script, it should use Babel to transpile the JavaScript code inside of the src folder and write it to a folder called lib.
Test it!
Type npm run build (This runs the build script in package.json)
This will create a new folder called lib and Babel will write the ES5 code to a file named TranspileExampleScript.js (it’s always the same name as the original file)
Note: This example will transpile all JavaScript files inside of the src folder. This is helpful as you build larger JavaScript projects — regardless of the number of JavaScript files, you only need to run one command (npm run build) to transpile all of your code.
Open up the newly created JavaScript file to see the transpiled code like the image below.
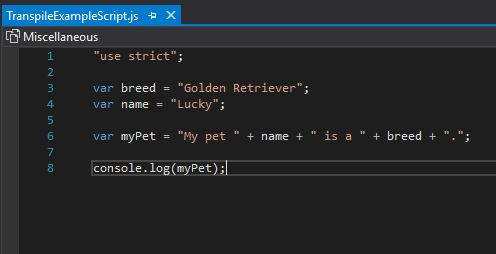
You should see that it looks very similar to the code I transpiled at the beginning of this guide except for the first line. The purpose of "use strict" is to indicate that the code should be executed in "strict mode". With strict mode, you can not, for example, use undeclared variables. All modern browsers support "use strict" except Internet Explorer 9 and lower:
Please Share and Comment. I love feedback.
Click here to check out more guides.
Thank you for checking out this technical guide on Transpiling JavaScript ES6+ to ES5.
Comments